Taking Off with Golang: An Introduction to the Corellium API Bindings for Go
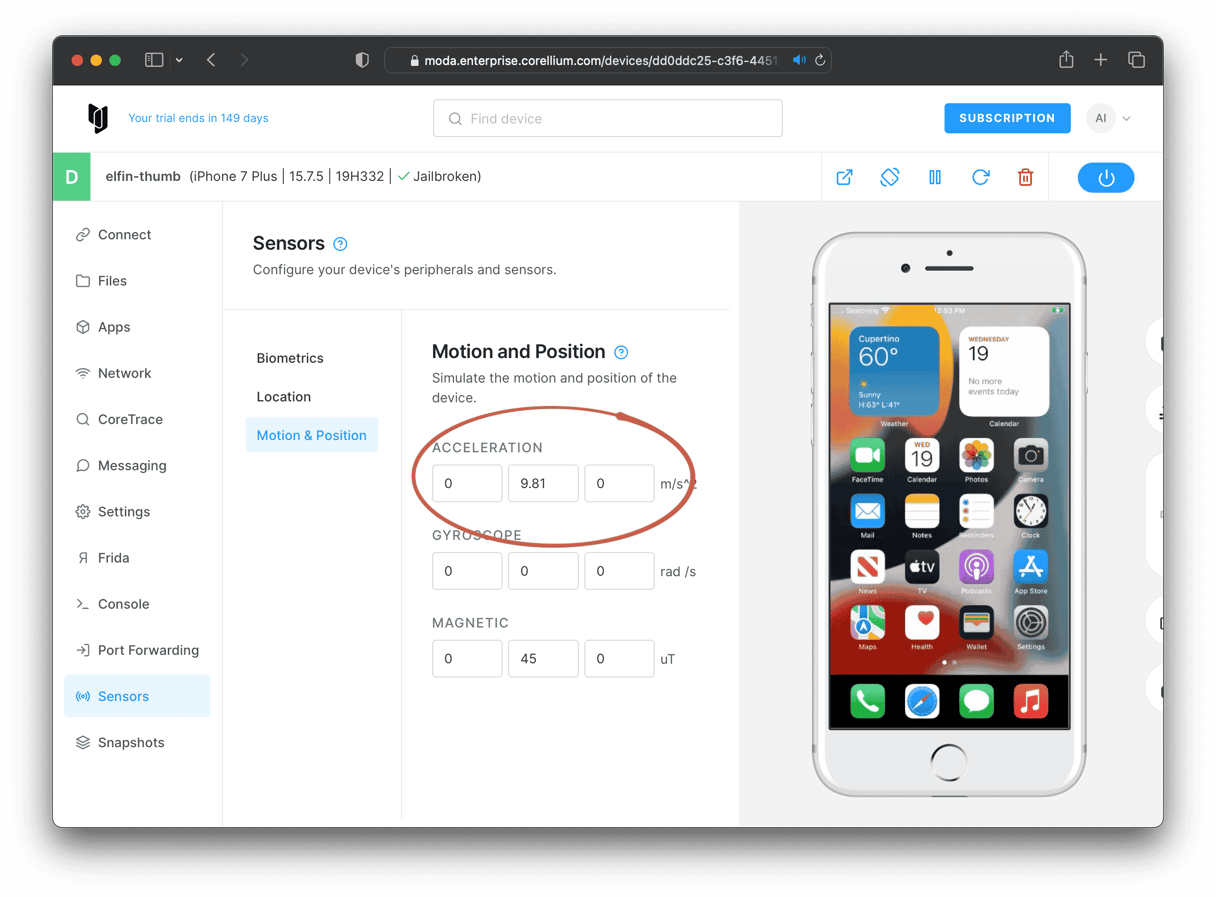
Golang, or simply Go, has rapidly gained popularity due to its simplicity, efficiency, and strong support for concurrent programming. In this blog post, we will demonstrate how to use the new Corellium API bindings for Golang to simulate changing the accelerometer of a virtual iPhone as if it was in the pocket of a passenger during a 737 takeoff.
This example showcases the power of Go combined with the capabilities of the Corellium API to turn these boring static fields into dynamically changing values to simulate the real world.
Writing The Program
Importing Required Libraries
First, we import the necessary libraries, including the Corellium API bindings, os, fmt, and time packages:
import (
"context"
"fmt"
"os"
"time"
corellium "github.com/aimoda/go-corellium-api-client"
)
Initializing Variables and Configuration
In the main function, we initialize the API token, context, peripherals data, instance ID, and API configuration using the Corellium API bindings for Golang:
apiToken := os.Getenv("CORELLIUM_API_TOKEN")
auth := context.WithValue(context.Background(), corellium.ContextAccessToken, apiToken)
peripheralsData := *corellium.NewPeripheralsData()
instanceId := "dd0ddc25-c3f6-4451-836f-4594fe00181f"
configuration := corellium.NewConfiguration()
configuration.Host = "moda.enterprise.corellium.com"
apiClient := corellium.NewAPIClient(configuration)
Simulating 737 Takeoff Acceleration with a Goroutine
We create a goroutine to simulate the 737 takeoff acceleration. In this goroutine, we use a ticker to trigger events every 500 milliseconds and iterate through the acceleration profile:
go func() {
ticker := time.NewTicker(500 * time.Millisecond)
defer ticker.Stop()
accelerationProfile := []float32{0.5, 1.0, 1.5, 2.0, 2.5, 2.7, 2.8, 2.9, 2.95, 2.95}
for _, accelerationX := range accelerationProfile {
<-ticker.C
accelerationY := float32(0.0)
accelerationZ := float32(0.0)
peripheralsData.SetAcceleration([]float32{accelerationX, accelerationY, accelerationZ})
resp, r, err := apiClient.InstancesApi.V1SetInstancePeripherals(auth, instanceId).PeripheralsData(peripheralsData).Execute()
if err != nil {
fmt.Fprintf(os.Stderr, "Error when calling `InstancesApi.V1SetInstancePeripherals``: %v\n", err)
fmt.Fprintf(os.Stderr, "Full HTTP response: %v\n", r)
}
fmt.Fprintf(os.Stdout, "Response from `InstancesApi.V1SetInstancePeripherals`: %v\n", resp)
}
}()
Waiting for the Goroutine Finish
Finally, we wait for the goroutine to finish by using a time.Sleep(). Our completed program should look like this:
package main
import (
"context"
"fmt"
"os"
"time"
corellium "github.com/aimoda/go-corellium-api-client"
)
func main() {
apiToken := os.Getenv("CORELLIUM_API_TOKEN")
auth := context.WithValue(context.Background(), corellium.ContextAccessToken, apiToken)
peripheralsData := *corellium.NewPeripheralsData() // PeripheralsData | New peripherals state
instanceId := "dd0ddc25-c3f6-4451-836f-4594fe00181f" // string | Instance ID
configuration := corellium.NewConfiguration()
configuration.Host = "moda.enterprise.corellium.com"
apiClient := corellium.NewAPIClient(configuration)
// Goroutine to simulate 737 takeoff acceleration
go func() {
ticker := time.NewTicker(500 * time.Millisecond)
defer ticker.Stop()
// Acceleration profile based on a simplified 737 takeoff
accelerationProfile := []float32{0.5, 1.0, 1.5, 2.0, 2.5, 2.7, 2.8, 2.9, 2.95, 2.95}
for _, accelerationX := range accelerationProfile {
<-ticker.C
accelerationY := float32(0.0)
accelerationZ := float32(0.0)
peripheralsData.SetAcceleration([]float32{accelerationX, accelerationY, accelerationZ})
resp, r, err := apiClient.InstancesApi.V1SetInstancePeripherals(auth, instanceId).PeripheralsData(peripheralsData).Execute()
if err != nil {
fmt.Fprintf(os.Stderr, "Error when calling `InstancesApi.V1SetInstancePeripherals``: %v\n", err)
fmt.Fprintf(os.Stderr, "Full HTTP response: %v\n", r)
}
// response from `V1SetInstancePeripherals`: PeripheralsData
fmt.Fprintf(os.Stdout, "Response from `InstancesApi.V1SetInstancePeripherals`: %v\n", resp)
}
}()
// Wait for the goroutine to finish
time.Sleep(10 * time.Second)
}
Runway Test
Finally, to test what we wrote, set the CORELLIUM_API_TOKEN to the value obtained from your profile, and run go run main.go.
And you’ve taken off!
Keep reading
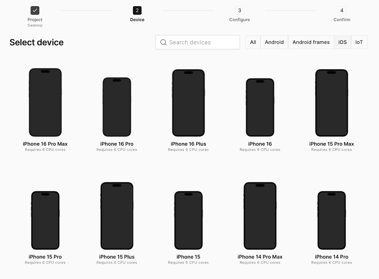
Meeting Compliance Without Compromise: Why Virtual Testing Is the Fastest Path to Regulatory Confidence and Faster Releases
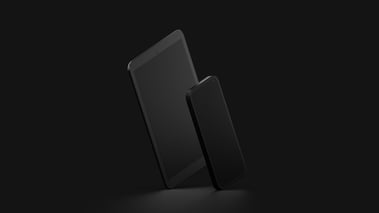
Mobile Application Development Isn’t Just for iPhones - Enable More Through iPad App Testing
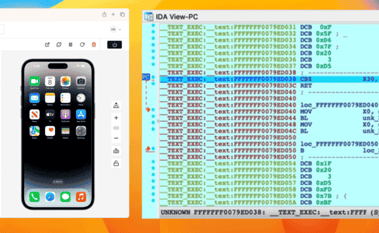